views
Mastering Component Testing in Angular with Jest: A Comprehensive Guide
In modern web development, ensuring that your application is functional, bug-free, and efficient is more crucial than ever. Angular, a widely adopted framework, has built-in tools for testing various aspects of an application. However, when it comes to component testing Angular applications, developers often seek the best practices and tools to streamline the process. In this comprehensive guide, we’ll walk you through component testing in Angular using Jest, providing a complete understanding of the process, common challenges, and how to overcome them. We’ll also introduce powerful tools like Testomat.io, which can make the testing process significantly easier and more effective.
Why Component Testing Matters in Angular?
Component testing is essential for any Angular application because it helps ensure that the individual building blocks of your application function as expected. Components in Angular often contain both the UI and the business logic. Testing them ensures that they interact correctly with the services, UI elements, and other components. Without effective testing, errors in a component could affect the user experience and ultimately harm the application’s reliability.
By focusing on testing Angular components, developers can catch bugs early in the development process, leading to higher-quality applications and more efficient workflows. Component testing in Angular often requires testing the interaction between the component and its template, as well as how the component integrates with the Angular lifecycle hooks.
What Makes Jest Ideal for Angular Component Testing?
Jest is a popular testing framework known for its speed, ease of use, and rich feature set. Originally designed for testing React applications, Jest has evolved and become a powerful tool for testing JavaScript applications in general, including Angular. Let’s explore why Jest is a top choice for component testing in Angular:
-
Snapshot Testing: Jest's snapshot testing capability makes it easy to test component rendering. This is particularly useful for checking if changes to the component affect its output in unexpected ways.
-
Mocking Capabilities: Jest comes with built-in support for mocking dependencies, which is incredibly useful in testing components that rely on services or external APIs.
-
Zero Configuration: Jest works out of the box with minimal configuration, making it an attractive choice for developers who want to quickly set up a testing environment without spending a lot of time configuring the test suite.
-
Parallel Test Execution: Jest runs tests in parallel, making it faster than many traditional testing frameworks. This can significantly reduce the amount of time spent waiting for test results.
How to Get Started with Component Testing in Angular Using Jest
To begin testing Angular components with Jest, you need to set up a few things in your Angular project. Below is an overview of the essential steps to get started.
1. Install Jest and Required Dependencies
First, you’ll need to install Jest and the necessary dependencies. This includes the jest-preset-angular
package, which provides the necessary configuration for running Jest with Angular.
Run the following command to install Jest and its dependencies:
npm install --save-dev jest jest-preset-angular @angular-builders/jest
After the installation is complete, update the angular.json
file to use the Jest builder instead of the default Karma test runner.
2. Configure Jest in Your Angular Project
Next, configure Jest by creating a jest.config.js
file at the root of your project. This file will specify the necessary settings for Jest to work with Angular.
Here’s an example of a simple Jest configuration for Angular:
module.exports = {
preset: 'jest-preset-angular',
setupFilesAfterEnv: ['<rootDir>/src/test-setup.ts'],
testPathIgnorePatterns: ['<rootDir>/node_modules/', '<rootDir>/dist/'],
transformIgnorePatterns: ['node_modules/(?!@ngrx)'],
};
This configuration ensures that Jest correctly handles Angular-specific setups like component templates and TypeScript files.
3. Write Your First Component Test
Now, you’re ready to start writing tests for your Angular components. Let’s assume we have a simple AppComponent
that needs to be tested.
Create a file called app.component.spec.ts
in the same directory as your component. This file will contain the test code for your component.
Here’s an example test for AppComponent
:
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { AppComponent } from './app.component';
describe('AppComponent', () => {
let fixture: ComponentFixture<AppComponent>;
let component: AppComponent;
beforeEach(() => {
TestBed.configureTestingModule({
declarations: [AppComponent],
});
fixture = TestBed.createComponent(AppComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create the component', () => {
expect(component).toBeTruthy();
});
});
This test simply checks if the AppComponent
is created successfully. More complex tests can check if certain elements are rendered, if user interactions work as expected, and if the component behaves correctly with mocked data.
4. Running the Tests
To run your Jest tests, use the following command:
npm test
Jest will run all the tests in your project, outputting the results in the terminal. If any tests fail, Jest provides helpful error messages that make it easier to debug the issue.
Overcoming Common Challenges in Component Testing with Jest
While Jest simplifies component testing, developers may face certain challenges when testing Angular components. Let’s explore some common issues and how to resolve them:
-
Handling Asynchronous Code: Angular components often interact with APIs or other asynchronous services. To test components with asynchronous behavior, use
fakeAsync
andtick
from Angular’s testing utilities to simulate the passage of time and handle asynchronous operations. -
Mocking Services: When testing components that depend on Angular services, you’ll need to mock those services. Jest provides built-in mocking functionality that allows you to easily mock service methods and return fake data.
-
Testing Input and Output Bindings: Angular components often rely on
@Input
and@Output
properties for passing data and communicating with other components. Jest makes it easy to simulate these bindings by setting the values directly in your test code and verifying that the component reacts correctly.
Tools That Can Enhance Your Angular Component Testing
In addition to Jest, several tools can further improve the quality of your testing process. Here are a few tools that you may find helpful for component testing in Angular:
-
Testomat.io: A powerful test management platform that allows you to track and organize your Jest tests, view test results, and manage test case scenarios.
-
Jest: A fast and easy-to-use testing framework that supports Angular testing with minimal configuration.
-
Karma: Although Jest is becoming more popular, Karma is still widely used for Angular testing. It works well in conjunction with Angular CLI.
-
Cypress: An end-to-end testing tool that provides a complete testing environment for your Angular applications.
-
Protractor: Angular’s end-to-end testing framework, though it is gradually being replaced by other tools like Cypress.
By integrating these tools into your testing workflow, you can further enhance your ability to write, manage, and execute high-quality tests.
Testomat.io: Streamlining Your Component Testing with Advanced Features
Testomat.io is a powerful test management tool that can make your Angular component testing workflow more efficient. It provides a platform where you can track test cases, generate reports, and visualize test results. Here’s how Testomat.io can help streamline your component testing process:
-
Centralized Test Management: Testomat.io allows you to manage all your tests in one place, making it easier to track progress and ensure that your Angular components are thoroughly tested.
-
Detailed Reports: With Testomat.io, you can generate detailed test reports that provide insights into your test coverage and results.
-
Integration with Jest: Testomat.io integrates seamlessly with Jest, enabling you to manage your tests, analyze results, and improve your testing processes.
To get started with Testomat.io and improve your component testing workflow, visit the official site and explore the various features that can enhance your testing practices.
Conclusion: Efficient Angular Component Testing with Jest and Testomat.io
Component testing Angular applications with Jest is a powerful way to ensure the quality and reliability of your app. By following best practices and leveraging tools like Testomat.io, you can streamline your testing process, reduce errors, and enhance the overall performance of your applications.
If you want to dive deeper into Angular component testing with Jest, be sure to check out the full guide and learn how to implement effective testing strategies. Additionally, Testomat.io offers a robust platform to manage and track your testing efforts, ensuring your Angular applications are fully optimized and bug-free.
For more information on component testing with Angular and Jest, visit the official Testomat.io blog.
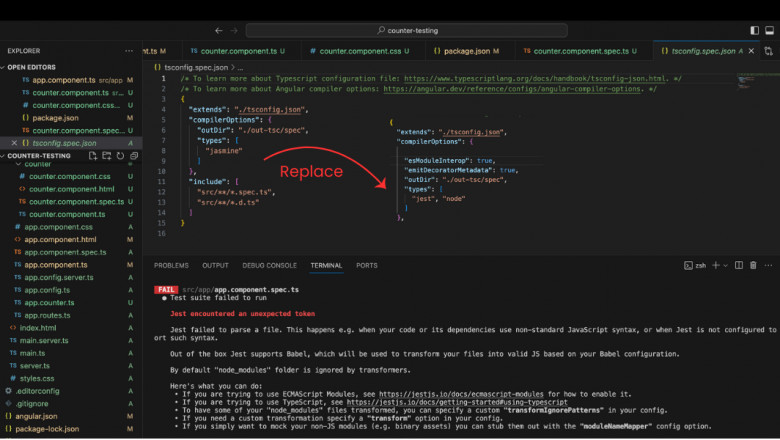

Comments
0 comment